Running V8 – The Heart of Node.js
What is V8?
The V8 JavaScript engine is like a super-fast translator for computers. When you write code in JavaScript, computers can’t understand it directly. V8’s job is to instantly convert that JavaScript into a language the computer can understand (machine code), so programs run quickly and smoothly.
It’s best known for powering Google Chrome and Node.js. Think of it as the engine in a car—without it, JavaScript just wouldn’t “go.” V8 turns human-friendly JavaScript into computer-friendly instructions, really fast.
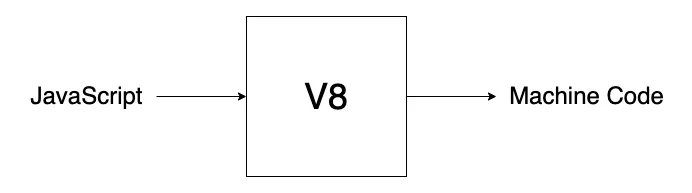
In this post, we will download, install, and run V8. We’ll also execute a simple JavaScript script using it.
Step 1: Install Dependencies
Before building V8, install the required dependencies.
For MacOS:
brew install python3 ninja llvm
For Ubuntu/Debian:
sudo apt update
sudo apt install -y build-essential python3 clang lldb lld ninja-build
Step 2: Clone Depot Tools
git clone https://chromium.googlesource.com/chromium/tools/depot_tools.git
echo 'export PATH="$PWD/depot_tools:$PATH"' >> ~/.zshrc # Add to PATH .bashrc or zshrc
source ~/.zshrc # Apply changes
Step 3: Fetch V8 Source Code and Sync
fetch v8
cd v8
gclient sync
Step 4: Build V8 (this take a while)
tools/dev/gm.py x64.release
Step 5: Running JavaScript with V8
Create a test file, script.js,
const hello = 'Hello'
const v8 = 'v8'
console.log(`${Date()}: ${hello} ${v8}!`)
Run it with V8,
out/x64.release/d8 script.js
You should see an output in the terminal,
mayur@MacBook-Pro NodeJS % v8/out/x64.release/d8 script.js
Tue Feb 18 2025 16:41:33 GMT+0530 (India Standard Time): Hello v8!
This confirms that V8 is executing JavaScript directly.
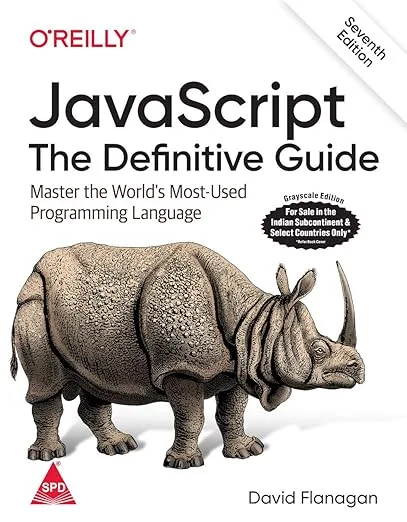
Conclusion
We’ve successfully learned how V8 compiles JavaScript code into machine code. However, it’s important to note that V8 itself only focuses on running JavaScript. It doesn’t have access to system resources like files, memory, or network calls, which are necessary for building full-fledged applications.
To interact with the operating system (OS) and make system calls (like reading files or handling network requests), we need to embed V8 in a C++ program. This C++ program can expose these OS-level system calls to JavaScript, allowing it to access the resources needed for more complex tasks. Essentially, V8 does the “brainwork” by running JavaScript, but C++ bridges the gap to the OS, enabling full application functionality.
In the next part, we’ll see how this integration works when we start building our own Node.js-like environment.
Let me know if you want to tweak anything! 🚀